Basic sphere («Hello World» example)
Creating a basic sphere that falls down on the plane.
To create whitestorm.js app you should make a basic HTML document with html
, head
and body
tags. Next step is to include Whitestorm.js to the document and main app script file. You can do it simply using script
tag.
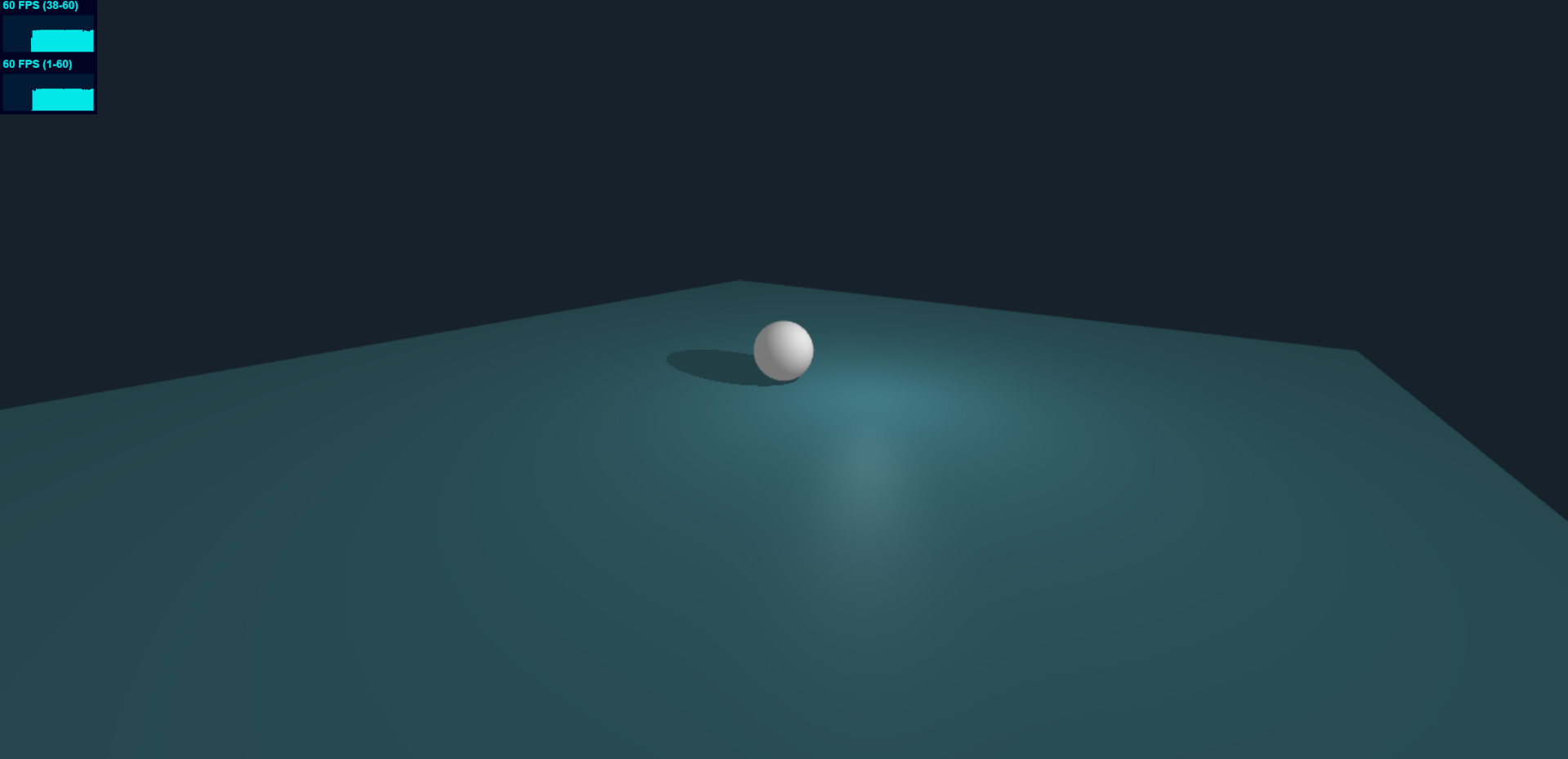
Try this helloworld demo online.
App
The first thing you should setup is the App object. When you do this, you do multiple things at once:
- Setup
THREE.Scene
- Make a perspective camera and add it to the scene.
- Apply background and other renderer options.
- Set auto resize (in addition).
const app = new WHS.App([
new WHS.app.ElementModule(),
new WHS.app.SceneModule(),
new WHS.app.CameraModule({
position: new THREE.Vector3(0, 0, 50)
}),
new WHS.app.RenderingModule({bgColor: 0x162129}),
new WHS.app.ResizeModule()
]);
You can also use a preset of modules
App
has it's own preset for simple apps quickstart.
Why is the color in Hexadecimal format?
Simply following Three.js' best practice.
const app = new WHS.App(
new WHS.BasicAppPreset() // setup for :
// ElementModule
// SceneModule
// CameraModule
// RenderingModule
);
Sphere
Next thing is a sphere. We define it's geometry in the following parameter. It has radius
, widthSegments
and heightSegments
- all of them are not required in WhitestormJS and have their defaullt values. material
is the following THREE.Material
instance that should be used.
// const app = ...
const sphere = new WHS.Sphere({ // Create sphere component.
geometry: {
radius: 3,
widthSegments: 32,
heightSegments: 32
},
material: new THREE.MeshBasicMaterial({
color: 0xF2F2F2
}),
position: [0, 10, 0]
});
sphere.addTo(app); // Add sphere to world.
Plane
And the last thing, add the plane and start rendering:
// const app = ...
// const sphere = ...
new WHS.Plane({
geometry: {
width: 100,
height: 100
},
material: new THREE.MeshBasicMaterial({
color: 0x447F8B
}),
rotation: {
x: - Math.PI / 2
}
}).addTo(app);
app.start(); // Start animations and physics simulation.
Updated over 7 years ago